React on Phoenix
8/Sep 2016
Phoenix’s author Chris McCord decided that instead of implementing a brand new pipeline for JavaScript and CSS, it was wiser to rely on existing tools. Specifically, Brunch was chosen.
An advantage of this is that using React with ES6 and JSX is dead easy, as Babel is already available for us.
- In your Phoenix project’s directory install the Node modules you’ll need:
$ npm install --save react react-dom babel-preset-react
- Change
brunch-config.js
and add thepresets
:
// Configure your plugins
plugins: {
babel: {
presets: ["es2015", "react"],
// Do not use ES6 compiler in vendor code
ignore: [/web\/static\/vendor/]
}
},
- Add a div for your React in your view…
<div id="app"></div>
- … and React code in
app.js
import React from "react"
import ReactDOM from "react-dom"
class Title extends React.Component {
render() {
return (<h1>React works!</h1>)
}
}
ReactDOM.render(
<Title />,
document.getElementById("app")
)
Et voila!
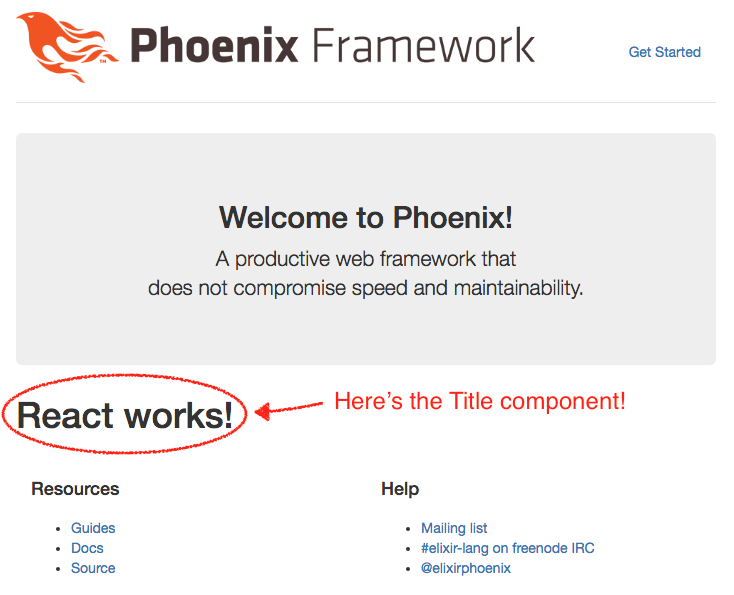
Have fun!